So Christmas is right around the corner and I was helping put up the decorations. One decoration in particular were some window lights that have multiple settings, you can control the settings via a switch on the cable or you can download an app and connect to it via bluetooth. Pretty nifty.
Our tree on the other hand is on a smart plug, which I can control via google assistant. Since google assistant can chain commands now it’s pretty easy to just tell her to “Let it snow” and she’ll turn on the tree, dim the lights, start playing a fireplace video on the TV and play my Christmas playlist on spotify – but those pesky lights in the corner won’t switch on til I boot up the app, start my bluetooth and connect. This would obviously not do – and I had to set about trying to bridge the gap.
The first thing to note with this is that Google Assistant doesn’t have an SDK (unlike Alexa which has events in AWS) so you’re a little limited. What I basically wanted was a simple command, such as “Hey Google, Switch on the window Lights” and have the window lights come on. So I did some research and found that although there is no SDK – there is an online service named IFTTT (If This Then That) where you can hook into google assistant. So in order to activate my lights I’d need to write an IFTTT applet.
The plan was simple. I’d write an applet that would send a signal to my Raspberry Pi – I would then write a script on my Pi that would make use of the bluetooth radio to contact the lights, I knew this could be done through python so I performed the actions on my phone, snooped the bluetooth actions and recreated them. Now all I needed was a trigger.
Applets are written throught the IFTTT Platform, The platform has an enterprise license and a personal license – so producing an applet for myself was free. I created as service for myself and went to Applets to produce one.
Clicking new applet the process was fairly straightforward, an applet is made up of a Trigger and then one or more Actions, so our Trigger we knew was google assistant. Once Selected you have a few choices to build your command structure, I went with a simple command and text ingredient, this is the phrase you say after “Hey Google” and the dollar sign $ is a wildcard of what you say afterwards, so I simply set mine to
Pi Function $
I chose Pi Function because for some reason my assistant struggled to make out “custom function” and kept thinking I was after info on Canoes. After you’ve set up a command format you can tell it to say something back to you and that’s the trigger all set up.
The action is a little more complicate, I figured the easiest thing to do for my Pi would be to get an API hit. I could host a php page fairly easily and my IP is fairly static, enough for Christmas at least. So I wanted something that would send a web request.
After searching the available Triggers I happened upon the Webhooks service, which sends a formatted request to any endpoint you like. When you set up the Webhook you can set the fields to be static values, or let your users select their own when you come to set up the applet. I elected the latter option as even though this was private, I didn’t want to list my endpoint explicitly.
The only ‘gotcha’ is passing the text ingredient – IFTTT won’t let you use the text ingredient unless it’s explicitly set in the applet settings, so for the content type and body of the webhook I set a JSON request including the text ingredient.
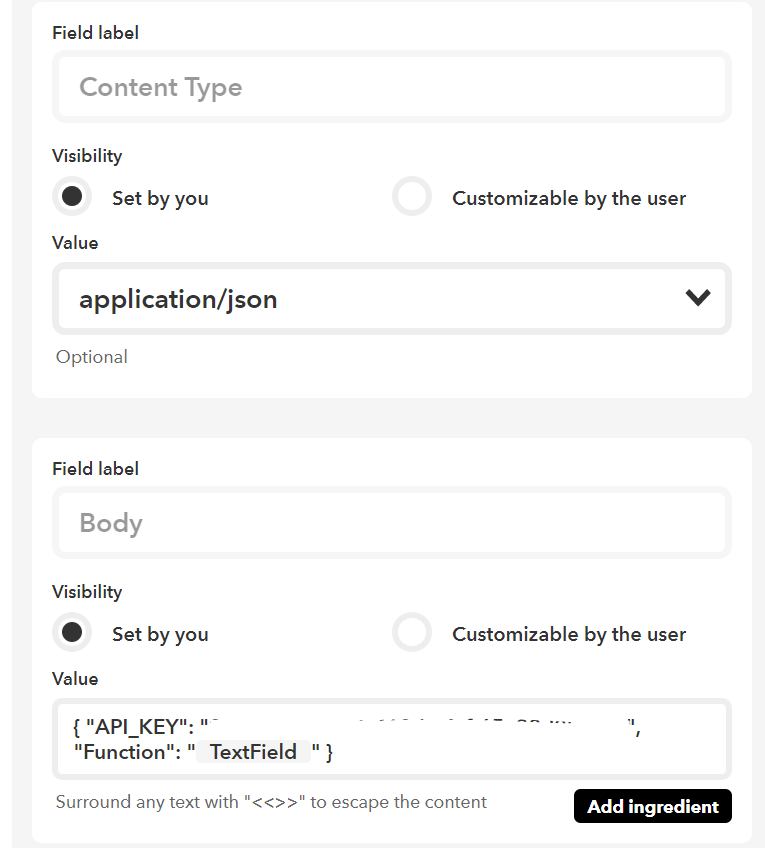
So as you can see I’m sending a JSON Post request to my endpoint, including a secure key so others can’t just spam my endpoint – and passing in a function param which is the text of the function I want to run.
Once the applet has been created – you should have the option to active the applet against your account. You may need to allow IFTTT to have some permissions against your Google account, but once added you should be able to set the correct endpoint.
Now all I had to do was write a PHP script to pick it all up. I installed apache, secured the connection with certbot and wrote a few scripts. I was essentially going to be making use of the php shell_exec function, so I just needed some scripts in the web dir to get me started. One of the important things was also writing a script to log the requests that came in, just in case I did start getting some abuse. My api.php looked like this
<?php
ini_set('display_errors', 1);
ini_set('display_startup_errors', 1);
error_reporting(E_ALL);
header("Content-Type: application/json; charset=utf-8");
// Takes raw data from the request
$json = file_get_contents('php://input');
$data = json_decode($json);
if ($data->API_KEY == "OBFUSCATED FOR REASONS") {
//Log the request
$IP = $_SERVER['REMOTE_ADDR'];
$Function = $data->Function;
$output = shell_exec("./LogRequest.sh ".$IP." ".$Function);
//Now we need to run out request
$function = strtolower($Function);
if ($function == "restart" || $function == "reboot")
{
shell_exec("./restart.sh");
}
else if ($function == "kodi")
{
shell_exec("./kodi.sh");
}
else if ($function == "lights")
{
shell_exec("python ./lights.py");
}
}
?>
Another GOTCHA you may find is that the text ingredient from IFTTT is for some reason limited to single words, this was a pain but not impossible – because google can indeed chain commands so you can link a weird command like “hey google, turn on the window lights” and make it mean “hey google, pi function lights”

The most interesting thing for me, as you may have noticed in my script, is that you don’t have to stop there – with an API hook on a Raspberry Pi – a lot more of the world is available to you, I added commands such as Reboort or Kodi so that when there’s an issue and I can just do these actions without having to pick up the remote.
I honestly believe that through shell scripts you can accomplish a lot – and with this tech in place I can make
Recent Comments